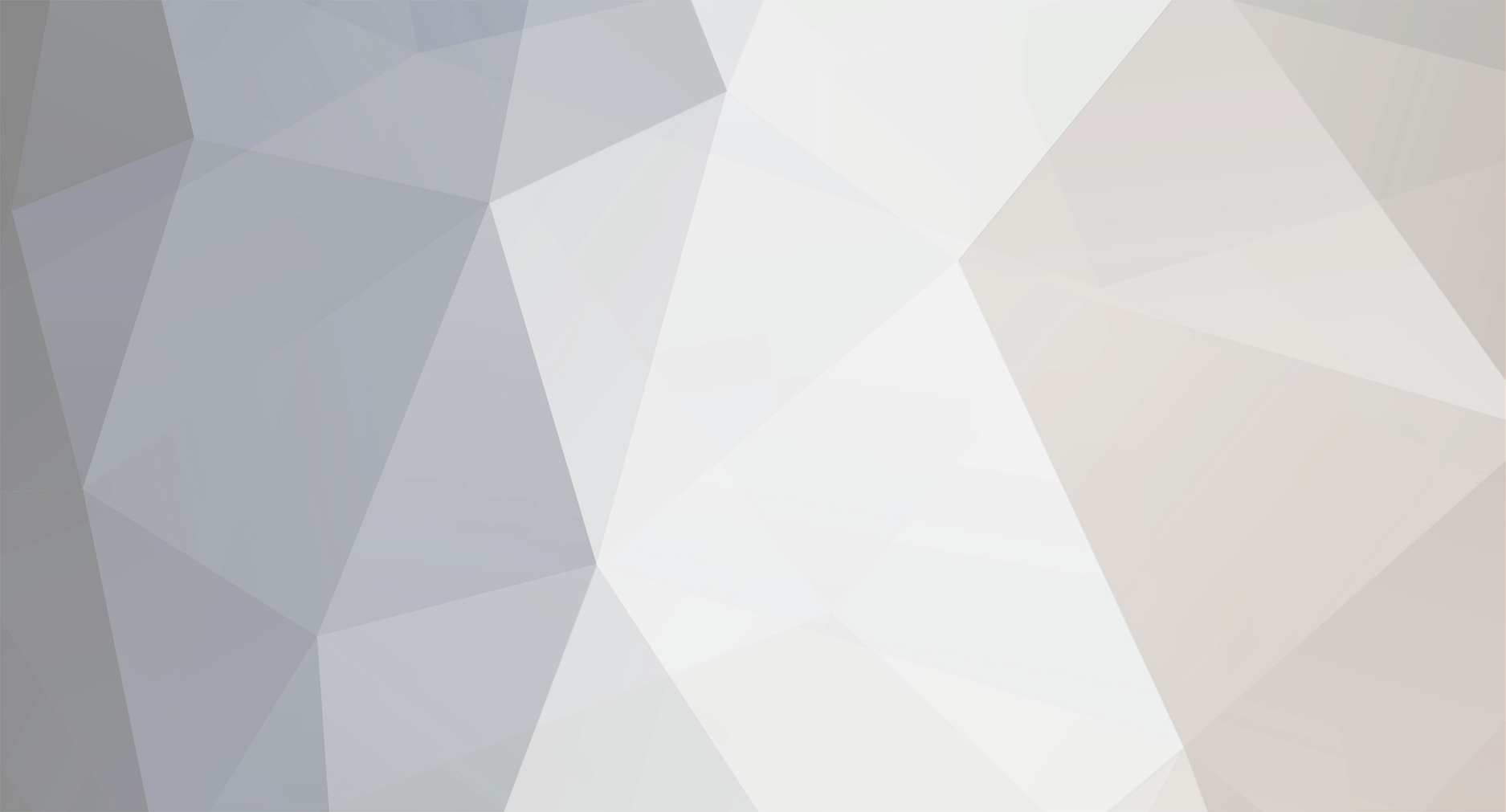
TheAxeMan33
-
Posts
237 -
Joined
-
Last visited
-
Days Won
5
Content Type
Profiles
Forums
Calendar
Tutorials
Downloads
Gallery
Posts posted by TheAxeMan33
-
-
There are modifications that pack their mods with a packer provided by EM4 / Sixteen Tons Entertainment. BUT, Only few modifications feature this option, like the London Multiplayer Modification. People use win-rar because it's compressed, and it takes less to download and upload due to it being compressed. If a file / modification is titled like modificationname.e4mod, then you can unpack it using the unpacker / modinstaller provided.
-
3 hours ago, TheAxeMan33 said:
After it does it's diagnostic save the information and upload it.
-
On 6/13/2016 at 6:31 PM, 59955_1453496446 said:
I have no clue
3).Get your DirectX Diagnostic Log
Start:
Run:
Type in dxdiag and press ok.
After it does it's diagnostic save the information and upload it.
-
What specifications does your computer have? Both modifications (even the SD version) are very power-hungry due to the realistic graphics.
-
9 minutes ago, ThatOneIowan said:
Link doesn't go anywhere to download
I think that the download was not approved yet, it usually takes a little for NFK to review it fully.
-
4 hours ago, odenker said:
The Bieberfelde Mod as it turns out has many viruses in it. Now I have removed the malware and trojans, but no games (including EM4) will launch. I try to run EM4, get a black screen, and then it crashes.
It has no viruses, if your game is crashing when loading the mod, it's strictly the fact that it cannot handle your computers specifications. The modification is highly detailed, and which makes mods require more power and space, like Manhattan Modification and some others. Emergency Planet would not allow the modification to have a page here if they thought it would pose a danger to the Community.
-
11 hours ago, cgibson2 said:
I have a question regarding changing parameters. We changed them to take out anything that would be related to ems and fire, but instead of disabling the events, they were multiplied it seemed. We changed first in endless and then endless_mp and then endless_2_mp... no change to the game except calls happen more often and are all fire related. We were trying for just having police calls. What are we doing wrong? I know that both players have to have the same files and all, so we change them together. Am I changing parameters in wrong file? I would really like to play the game to how we want, love the map and all. Am I supposed to be changing events on challenge not endless? For single I always played endless so for that it was always the endless file I changed. Thanks again!!
Thanks for the help!! (hopefully someone can help me) Thanks again!!
Chris
If you could send me a message containing the changes you made (copy and pasting the freeplay parameters into the text box) so that I can assist you in private chat rather than have people think it's related to the modification. I'd be more than happy to assist you.
~ Axe
-
9 minutes ago, brandanl said:
Anyone know why the ambulances don't drop off patients at the hospital? They just sit there and do nothing, kind of a game breaking bug. Additionally, the ambulances at the hospital cannot move without being popped out one by one like a stack (one by the helipad).
I assume there was no beta-testing, so there are a lot of bugs, such as the to-hospital script not being rescripted, the script is told to get the 2 paramedics with the stretcher out, not to get the paramedic with the stryker script. It's a issue the mod author needs to fix, nothing that can be done on your end or that only you have. For the ambulances not moving in a group, it's just the fact that it's so cluttered with them in such a small dense space, you can send some off the map to fix that issue. If you want to continue playing, just send them all home and grab new ambulances, but this time choose to have a stretcher paramedic on the buy menu.
-
1
-
-
Theres no real "software" for downloading modifications, you can use the included modinstaller but that only works for .e4mod files. Normally they are packaged in a folder by WINRAR, which can be found HERE. If you still need help, go ahead and look at this video tutorial made by TheNorthernAlex.
-
4 hours ago, freakinfuzzball said:
Just had a crash to desktop when a call came in, no idea what happened but I didn't get an error message. Here's the logfile.
http://www.emergency-planet.com/applications/core/interface/file/attachment.php?id=17762
Start off with closing the area off, have units stop civilians from entering the demonstration zone and have a zone set for EMS and Fire to stage, once SWAT and the Riot Control squads arrive, spread them out or group them up, depending on how you prefer it. Get them into the transport vehicle as fast as possible, especially the ringleader, if you transported all the demonstrators then you should be finished.
-
On 4/24/2016 at 3:42 PM, 59955_1453496446 said:
Does anyone know who is the one with the Boston mod on the Manhattan mod map??
if I'm right Rafael made that along with some of the developers on his team. I don't think it ever got released, I know a-few have beta versions but you won't find it out for public downloading.
-- To FInn ; I get CTD when I call for the NYPD Rollback in Tech.
-
On 4/16/2016 at 4:57 PM, wolfdog240 said:
i download boston mod beta 2.0 it crash to desktop
I'd like to first start by welcoming you into our Community. I'l attach a post below made by Mikey that contains the full list of what we need to possibly be of some assistance to you. Please head to the Technical Support category and do the following in a new topic..
Quote1).Searched the Forum using the search tool located on the top menu.
2).Have your logfile from your game so that it may be reviewed.
EX: C:\Program Files\Wizardworks\Emergency 911 First Responders\Logfile.txt.
3).Get your DirectX Diagnostic Log
Start:
Run:
Type in dxdiag and press ok.
After it does it's diagnostic save the information and upload it.
4).List your system specs if the information of your hardware does NOT match the DX Diag or information was omitted from the report.
Please also remember to use the "Upload" file or "Wrap in Code Tags" options to attatch your DirectX Diagnostic log or your Logfile.txt so that they can be viewed without consuming a large quantity of a page. To upload the file, just browse and select the .txt file and press "UPLOAD" and they will be attatched. The alternative will produce a code box around your choosen text, preserving all coded information as it belongs. To do this you can hit the button found to the left of the "youtube" button and [code}{/code] (replace the }{ with ][) appears, copy and paste your logfiles into the space between the ][ symbols and post. Any post that has the log file directly written in the post without either the code box or attatch feature WILL Be edited/removed.
-
On 4/5/2016 at 11:05 AM, 999madtom said:
Sorry for bringing back a dying topic.
How do you turn off ambushes? I spent the last 10 minutes trying to find it.
C:\Program Files (x86)\sixteen tons entertainment\Emergency 4\Mods\US Army Mod\Scripts\Game\Mission
Incase you haven't found it already.
-
I'm attempting to integrate the script that makes the vehicle speed-up when the flashing lights or sirens are on. I do not know where to get the script from.
-
12 hours ago, Murf said:
I think I answered you before, it's a corona problem. Look around the forums instead of asking twice, but since I'm a nice guy I'll give it to you! In the link I provided below you'll find instructions on how to install it too.
http://www.moddb.com/mods/mayberry-mod-2014/addons/corona-update
Unexpected end of archive when it finishes downloading by the way, not sure if it's me or your upload.
I can confirm this at the end, I believe it might have something to do with the issue they previously had.
-
Hm, worked for me perfectly, but maybe that's because it's my first time voting on this poll, shows results and such.
-
3 hours ago, CFDDIVE11 said:
What are the containers for and what do they do?
Special Response Containers basically, they carry gear a normal rig wouldn't and are used during urgent times for special responses.
-
The current amount of hydrants make it realitic, you won't have a hydrant for every location, that's why we have tankers, to shuttle water.
-
3 hours ago, tbone1fire said:
yep I did the works. Updated everything and reinstalled everything and still the same outcome
PC Specs?
-
Do you have a download link mate
-
Hello out there this is the latest link for Boston Mod Beta thank you for your support
There is a read me letter for credits if anyone I missed please notify me I will update with other stuff in the future.
If you want to fix any of the bugs please do so and include it on the page for the members.
If you have any interest on working on the Boston mod, you have to have good experience in modding script work, mapping excreta. You will need promotion from me and the molders to make any chances or use the work to this mod Finn!
Happy New Year Please don't contact me with trouble questions this mod dose have bugs but is very playable for hours. As always thanks to the Emergency Planet Forum Sixteen Stones EM4 Hoppah LA Mod which is where is the sub mod is derived from. Link is also on the first page.
!3IxujWAWsyWaOxXmBvoDWfDJ2KOC6PC2N1V2Sg6Zojc
Thankyou so much for the link, was really hoping to get a game in tonight but I got bored of playing the same modifications, I'l give it a shot and beta test it for a good time and let you know some recommendations/additions that might be beneficial to the modification
-
We had a crash as of now, so hereby the logfiles requested;
http://www.uploader.gamergun.com/files/1/logfile.txt
http://www.uploader.gamergun.com/files/1/DxDiag.txt
- Copied text from squad55.com and couldn't figure out how to change the highlight color, so ye
Go ahead and submit a thread on http://forum.emergency-planet.com/forum/42-technical-related-support/ and supply the files and abit more information, any information that would provide a person the ability to diagnose your problem.
-
ofcourse, making us jealous as hell but so so worth it, the modification looks amazing, even just playing the .5 version is fun, it shows your units have a very unique design and complex and very overthought strategy.
-
This is a pretty big list of needed items and equipment / apparatus, it'd be best if you try yourself and then IF some people find you have your ideas set straight, then someone MIGHT help.
LAToPoliceStation Assistance
in Modding Related Support
Posted
//******************************************************************************************
// #Version 1.5#
//
// Includes: To police station command.
//
// - VcmdToPoliceStation
//
// Script by Hoppah
//
// Usage of this script in other mods is NOT allowed without permission of Hoppah
//
//******************************************************************************************
const char CMD_SIREN[] = "VcmdSiren";
const char CMD_AUTOSIREN_OFF[] = "VcmdAutoSirenOff";
const char CMD_WARNINGLIGHTS_OFF[] = "VcmdWarningLightsOff";
const char CMD_WARNINGLIGHTS_ON[] = "VcmdWarningLightsOn";
const char CMD_FLOODLIGHTS_OFF[] = "VcmdFloodLightsOff";
const char CMD_FLOODLIGHTS_ON[] = "VcmdFloodLightsOn";
const char CMD_GOHOME[] = "GoHome";
const char CMD_TOPOLICESTATION[] = "VcmdToPoliceStation";
const char CMD_PATROL[] = "VcmdPatrol";
const char CMD_STANDBY_ON[] = "VcmdStandbyOn";
const char CMD_STANDBY_OFF[] = "VcmdStandbyOff";
const char DUMMY_DISABLE[] = "DummyDisableSiren";
const char DUMMY_HASSIREN[] = "DummyHasSiren";
const char DUMMY_GOHOME[] = "DummyGoHome";
const char DUMMY_PATROL[] = "DummyPatrol";
const char OBJ_HELIPAD[] = "policestation_helipad";
const char VO_ENTRY[] = "policestation_entry";
const char VO_ENTRY_HELIPAD[] = "policestation_entry2";
const char VO_TURNTO[] = "policestation_turnto";
const char VO_PARK01[] = "policestation_park01";
const char VO_PARK02[] = "policestation_park02";
const char VO_PARK03[] = "policestation_park03";
const char VO_PARK04[] = "policestation_park04";
const char VO_PARK05[] = "policestation_park05";
const char VO_PARK06[] = "policestation_park06";
const char VO_HELI[] = "policestation_heli";
const char VO_SPAWN[] = "policestation_spawn";
const char SND_TOSTATION[] = "mod:Audio/FX/radio/1019.wav";
const char HINT_HELICOPTER[] = "Another helicopter is blocking the helipad! The helicopter will return to base!";
const char HINT_PD[] = "No more spots are available, starting patrol of sector.";
const char PROTO_CV_LAPD[] = "mod:Prototypes/Vehicles/03 LA Police/cv_lapd.e4p";
int DummyGroup = 32;
object VcmdToPoliceStation : CommandScript
{
VcmdToPoliceStation()
{
SetCursor("topolicestation");
SetIcon("topolicestation");
SetGroupID(DummyGroup);
SetGroupLeader(true);
SetRestrictions(RESTRICT_SELFEXECUTE);
}
bool CheckPossible(GameObject *Caller)
{
if (!Caller->IsValid())
return false;
if (!Game::IsFreeplay() && !Game::IsMultiplayer())
return false;
Vehicle v(Caller);
if (v.IsCollidingWithVirtualObject(VO_HELI))
return false;
ActorList l1 = Game::GetActors(VO_PARK01);
if (l1.GetNumActors() == 0)
return false;
return true;
}
bool CheckTarget(GameObject *Caller, Actor *Target, int childID)
{
if (!Caller->IsValid() || !Target->IsValid() || (Caller->GetID() != Target->GetID()))
return false;
Vehicle v(Caller);
SetPriority(0);
if (v.GetNumTransported() > 0)
SetPriority(110);
return true;
}
void PushActions(GameObject *Caller, Actor *Target, int ChildID)
{
Vehicle v(Caller);
bool Donut = false;
ActorList d = Game::GetActors(VO_PARK04);
if (StrCompare(v.GetPrototypeFileName(), PROTO_CV_LAPD) == 0 && d.GetNumActors() > 0)
Donut = true;
if(ChildID == 0)
{
if (v.GetVehicleType() != VT_POLICE_PHC)
{
if (v.IsBlueLightEnabled())
v.EnableBlueLights(false);
if (v.HasCommand("DummyFollow"))
v.RemoveCommand("DummyFollow");
if (v.HasCommand(CMD_STANDBY_OFF))
{
v.RemoveCommand(CMD_STANDBY_OFF);
v.AssignCommand(CMD_STANDBY_ON);
}
if (v.HasCommand(CMD_WARNINGLIGHTS_OFF))
{
v.EnableBlinker(BLT_NONE);
v.RemoveCommand(CMD_WARNINGLIGHTS_OFF);
v.AssignCommand(CMD_WARNINGLIGHTS_ON);
}
if (v.HasCommand(CMD_FLOODLIGHTS_OFF))
{
v.EnableSpecialLights(false);
v.RemoveCommand(CMD_FLOODLIGHTS_OFF);
v.AssignCommand(CMD_FLOODLIGHTS_ON);
}
if (v.HasObjectPath(NULL))
Game::ExecuteCommand(DUMMY_PATROL, &v, &v);
if (v.HasCommand(DUMMY_HASSIREN) && v.HasCommand(CMD_AUTOSIREN_OFF))
Game::ExecuteCommand(DUMMY_DISABLE, &v, &v);
bool ParkinglotFound = false;
bool ToDonut = false;
ActorList l1;
if (!ParkinglotFound)
{
GameObjectList l2;
Game::CollectObstaclesOnVirtualObject(VO_PARK01, l2, ACTOR_VEHICLE);
if(l2.GetNumObjects() == 0)
{
l1 = Game::GetActors(VO_PARK01);
ParkinglotFound = true;
}
}
if (!ParkinglotFound)
{
GameObjectList l3;
Game::CollectObstaclesOnVirtualObject(VO_PARK02, l3, ACTOR_VEHICLE);
if(l3.GetNumObjects() == 0)
{
l1 = Game::GetActors(VO_PARK02);
ParkinglotFound = true;
}
}
if (!ParkinglotFound)
{
GameObjectList l4;
Game::CollectObstaclesOnVirtualObject(VO_PARK03, l4, ACTOR_VEHICLE);
if(l4.GetNumObjects() == 0)
{
l1 = Game::GetActors(VO_PARK03);
ParkinglotFound = true;
}
}
if (!ParkinglotFound)
{
GameObjectList l5;
Game::CollectObstaclesOnVirtualObject(VO_PARK04, l5, ACTOR_VEHICLE);
if (l4.GetNumObjects() == 0)
{
l1 = Game::GetActors(VO_PARK04);
ParkinglotFound = true;
}
}
if (!ParkinglotFound)
{
GameObjectList l6;
Game::CollectObstaclesOnVirtualObject(VO_PARK05, l6, ACTOR_VEHICLE);
if (l4.GetNumObjects() == 0)
{
l1 = Game::GetActors(VO_PARK05);
ParkinglotFound = true;
}
}
if (!ParkinglotFound)
{
GameObjectList l7;
Game::CollectObstaclesOnVirtualObject(VO_PARK06, l7, ACTOR_VEHICLE);
if (l4.GetNumObjects() == 0)
{
l1 = Game::GetActors(VO_PARK06);
ParkinglotFound = true;
}
}
if (!ParkinglotFound)
{
v.PushActionExecuteCommand(ACTION_NEWLIST, CMD_PATROL, Caller, 0, false);
Mission::PlayHint(HINT_PD);
return;
}
else
{
Vector PD = l1.GetActor(0)->GetPosition();
Vector PD2 = l1.GetActor(0)->GetPosition() + Vector(280,-280,0);
ActorList l8 = Game::GetActors(VO_TURNTO);
Vector TurnTo = l8.GetActor(0)->GetPosition();
Game::FindFreePosition(Caller, PD);
Audio::PlaySample3D(SND_TOSTATION, v.GetPosition());
if (!ToDonut)
{
v.PushActionMove(ACTION_NEWLIST, PD);
v.PushActionTurnTo(ACTION_APPEND, TurnTo);
} else
{
v.PushActionMove(ACTION_NEWLIST, PD2);
v.PushActionTurnTo(ACTION_APPEND, PD + Vector(320,-320,0));
v.PushActionWait(ACTION_APPEND, 0.5f);
v.PushActionMove(ACTION_APPEND, PD);
}
v.PushActionWait(ACTION_APPEND, 1.5f);
v.PushActionExecuteCommand(ACTION_APPEND, CMD_TOPOLICESTATION, Caller, 2, false);
PersonList transports = v.GetTransports();
if (!ToDonut && transports.GetNumPersons() > 0)
v.PushActionExecuteCommand(ACTION_APPEND, CMD_TOPOLICESTATION, Caller, 1, false);
}
} else
{
Audio::PlaySample3D(SND_TOSTATION, v.GetPosition());
ActorList l1 = Game::GetActors(OBJ_HELIPAD);
if(l1.GetNumActors() > 0)
{
Actor policestation = *l1.GetActor(0);
Vector Policestation = policestation.GetPosition();
}
if (Game::IsSquadInVirtualObject(VO_HELI))
{
GameObjectList l2;
Game::CollectObstaclesOnVirtualObject(VO_HELI, l2, ACTOR_VEHICLE);
for (int i = 0; i < l2.GetNumObjects(); i++)
{
Vehicle m = l2.GetObject(i);
m.PushActionExecuteCommand(ACTION_NEWLIST, DUMMY_GOHOME, &m, 1, false);
Mission::PlayHint(HINT_HELICOPTER);
}
}
Game::FindFreePosition(Caller, Policestation);
GameObject obj(&policestation);
float landingDirection = v.GetValidLandingAngle(&obj, Policestation);
v.PushActionFlyTo(ACTION_NEWLIST, Policestation, true, landingDirection);
PersonList transports = v.GetTransports();
if (transports.GetNumPersons() > 0)
v.PushActionExecuteCommand(ACTION_APPEND, CMD_TOPOLICESTATION, Caller, 1, false);
}
}
if(ChildID == 1)
{
if (v.GetVehicleType() != VT_POLICE_PHC)
{
ActorList l1 = Game::GetActors(VO_SPAWN);
if (l1.GetNumActors() > 0)
Vector TargetPos = l1.GetActor(0)->GetPosition();
PersonList transports = v.GetTransports();
for(int i = 0; i < transports.GetNumPersons(); i++)
{
transports.GetPerson(i)->PushActionLeaveCar(ACTION_NEWLIST, Caller);
if(transports.GetPerson(i)->GetRole() == ROLE_GANGSTER)
{
transports.GetPerson(i)->SetRole(ROLE_CIVILIAN);
transports.GetPerson(i)->SetBehaviour(BEHAVIOUR_CIVILIAN_NORMAL);
}
transports.GetPerson(i)->PushActionWait(ACTION_APPEND, 0.5f);
transports.GetPerson(i)->PushActionMove(ACTION_APPEND, TargetPos, true);
transports.GetPerson(i)->PushActionDeleteOwner(ACTION_APPEND);
}
} else
{
ActorList l1 = Game::GetActors(VO_ENTRY_HELIPAD);
if (l1.GetNumActors() > 0)
Vector TargetPos = l1.GetActor(0)->GetPosition();
PersonList transports = v.GetTransports();
for(int i = 0; i < transports.GetNumPersons(); i++)
{
transports.GetPerson(i)->PushActionLeaveCar(ACTION_NEWLIST, Caller);
if(transports.GetPerson(i)->GetRole() == ROLE_GANGSTER)
{
transports.GetPerson(i)->SetRole(ROLE_CIVILIAN);
transports.GetPerson(i)->SetBehaviour(BEHAVIOUR_CIVILIAN_NORMAL);
}
transports.GetPerson(i)->PushActionWait(ACTION_APPEND, 0.5f);
transports.GetPerson(i)->PushActionMove(ACTION_APPEND, TargetPos, true);
transports.GetPerson(i)->PushActionDeleteOwner(ACTION_APPEND);
}
}
}
if(ChildID == 2)
{
Vehicle v(Caller);
v.EnableBlinker(BLT_NONE);
bool ToPoliceStation = false;
if (v.IsCollidingWithVirtualObject(VO_PARK01))
return;
else
ToPoliceStation = true;
if (v.IsCollidingWithVirtualObject(VO_PARK02))
return;
else
ToPoliceStation = true;
if (v.IsCollidingWithVirtualObject(VO_PARK03))
return;
else
ToPoliceStation = true;
if (v.IsCollidingWithVirtualObject(VO_PARK04))
return;
else
ToPoliceStation = true;
if (v.IsCollidingWithVirtualObject(VO_PARK05))
return;
else
ToPoliceStation = true;
if (v.IsCollidingWithVirtualObject(VO_PARK06))
return;
if(ToPoliceStation)
v.PushActionExecuteCommand(ACTION_NEWLIST, CMD_TOPOLICESTATION, Caller, 0, false);
}
}
};
I've been trying to get additional parking spots to work, because I find that the map is too big for me to keep track of my PD, as they always tend to create horrible traffic jams during big fire operations. I added the proper Virtual Objects into both freeplay maps, named them properly, added const char variables, etc. Park04-Park06 are the ones I added, Park01-Park03 were left unchanged and still work. The game seems to not recognize that there are more spots, I added in a hint system exactly like the helicopter one for when there is a heli on the pad already, and it's giving me the message that all spots are taken for Park04-Park06, but no objects are inside the objects or such. Any help would be much appreciated.