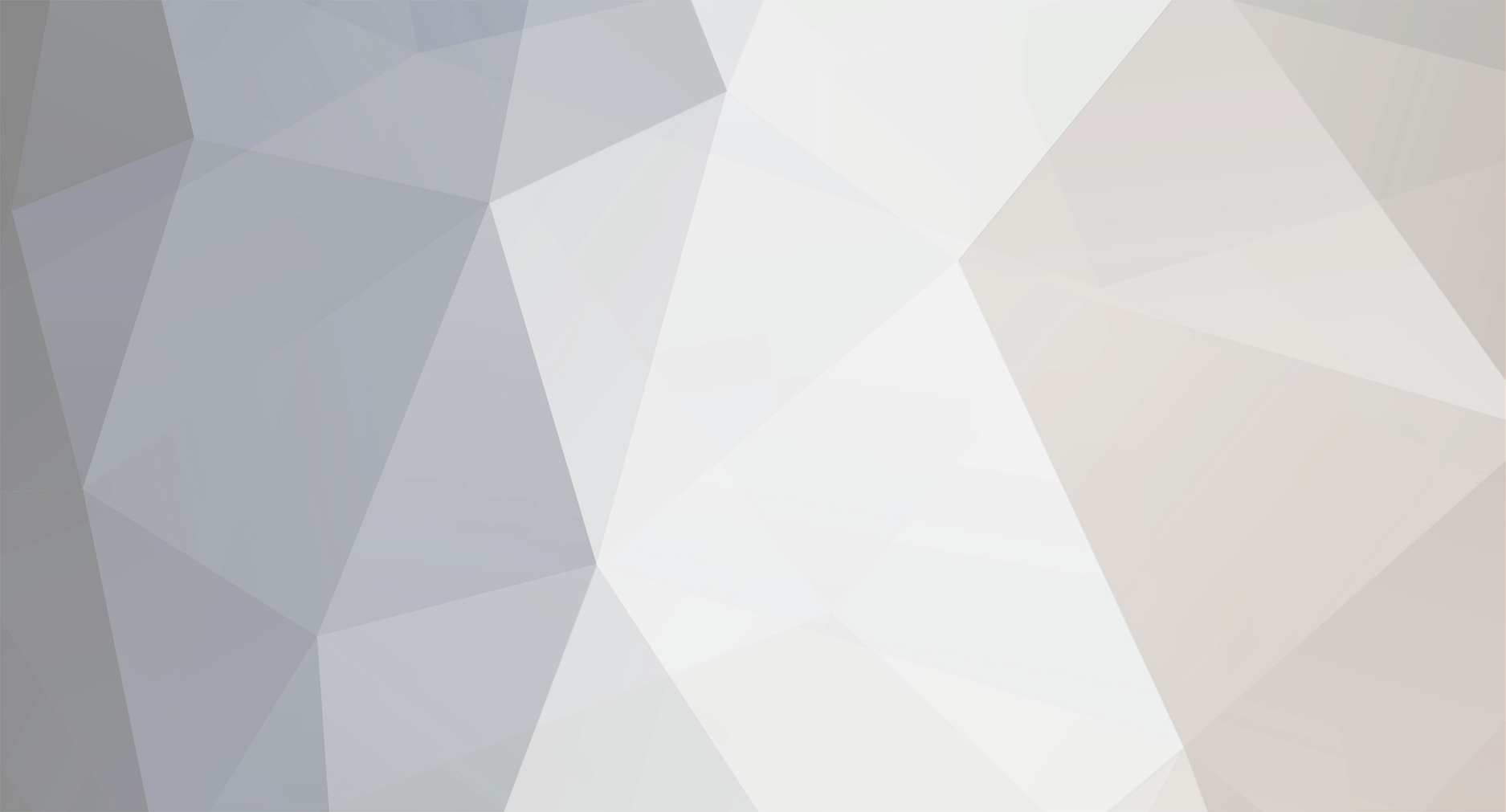
firefighter243
Members-
Posts
193 -
Joined
-
Last visited
Content Type
Profiles
Forums
Calendar
Tutorials
Downloads
Gallery
Everything posted by firefighter243
-
Here it is: //****************************************************************************************** // #Version 1.5# // // Includes: To police station command. // // - VcmdToPoliceStation // // Script by Hoppah // // Usage of this script in other mods is NOT allowed without permission of Hoppah // //****************************************************************************************** const char CMD_SIREN[] = "VcmdSiren"; const char CMD_AUTOSIREN_OFF[] = "VcmdAutoSirenOff"; const char CMD_WARNINGLIGHTS_OFF[] = "VcmdWarningLightsOff"; const char CMD_WARNINGLIGHTS_ON[] = "VcmdWarningLightsOn"; const char CMD_FLOODLIGHTS_OFF[] = "VcmdFloodLightsOff"; const char CMD_FLOODLIGHTS_ON[] = "VcmdFloodLightsOn"; const char CMD_GOHOME[] = "GoHome"; const char CMD_TOPOLICESTATION[] = "VcmdToPoliceStation"; const char CMD_PATROL[] = "VcmdPatrol"; const char CMD_STANDBY_ON[] = "VcmdStandbyOn"; const char CMD_STANDBY_OFF[] = "VcmdStandbyOff"; const char DUMMY_DISABLE[] = "DummyDisableSiren"; const char DUMMY_HASSIREN[] = "DummyHasSiren"; const char DUMMY_GOHOME[] = "DummyGoHome"; const char DUMMY_PATROL[] = "DummyPatrol"; const char OBJ_HELIPAD[] = "policestation_helipad"; const char VO_ENTRY[] = "policestation_entry"; const char VO_ENTRY_HELIPAD[] = "policestation_entry2"; const char VO_TURNTO[] = "policestation_turnto"; const char VO_TURNTO5[] = "fs_vogate05a"; const char VO_TURNTO6[] = "fs_vogate06a"; const char VO_PARK01[] = "policestation_park01"; const char VO_PARK02[] = "policestation_park02"; const char VO_PARK03[] = "policestation_park03"; const char VO_PARK04[] = "policestation_park04"; const char VO_PARK05[] = "policestation_park05"; const char VO_PARK06[] = "policestation_park06"; const char VO_PARK07[] ="policestation_park07", const char VO_PARK08[] ="policestation_park08", const char VO_PARK09[] ="policstation_park09", const char VO_PARK10[] ="policestation_park10", const char VO_PARK11[] ="policestation_park11", const char VO_PARK12[] ="policestation_park12", const char VO_PARK13[] ="policestation_park13", const char VO_HELI[] = "policestation_heli"; const char VO_SPAWN[] = "policestation_spawn"; const char SND_TOSTATION[] = "mod:Audio/FX/radio/1019.wav"; const char HINT_HELICOPTER[] = "Another helicopter is blocking the helipad! The helicopter will return to base!"; const char PROTO_CV_LAPD[] = "mod:Prototypes/Vehicles/03 LA Police/cv_lapd.e4p"; int DummyGroup = 32; object VcmdToPoliceStation : CommandScript { VcmdToPoliceStation() { SetCursor("topolicestation"); SetIcon("topolicestation"); SetGroupID(DummyGroup); SetGroupLeader(true); SetRestrictions(RESTRICT_SELFEXECUTE); } bool CheckPossible(GameObject *Caller) { if (!Caller->IsValid()) return false; if (!Game::IsFreeplay() && !Game::IsMultiplayer()) return false; Vehicle v(Caller); if (v.IsCollidingWithVirtualObject(VO_HELI)) return false; ActorList l1 = Game::GetActors(VO_PARK01); if (l1.GetNumActors() == 0) return false; return true; } bool CheckTarget(GameObject *Caller, Actor *Target, int childID) { if (!Caller->IsValid() || !Target->IsValid() || (Caller->GetID() != Target->GetID())) return false; Vehicle v(Caller); SetPriority(0); if (v.GetNumTransported() > 0) SetPriority(110); return true; } void PushActions(GameObject *Caller, Actor *Target, int ChildID) { Vehicle v(Caller); bool Donut = false; ActorList d = Game::GetActors(VO_PARK04); if (StrCompare(v.GetPrototypeFileName(), PROTO_CV_LAPD) == 0 && d.GetNumActors() > 0) Donut = true; if(ChildID == 0) { if (v.GetVehicleType() != VT_POLICE_PHC) { if (v.IsBlueLightEnabled()) v.EnableBlueLights(false); if (v.HasCommand("DummyFollow")) v.RemoveCommand("DummyFollow"); if (v.HasCommand(CMD_STANDBY_OFF)) { v.RemoveCommand(CMD_STANDBY_OFF); v.AssignCommand(CMD_STANDBY_ON); } if (v.HasCommand(CMD_WARNINGLIGHTS_OFF)) { v.EnableBlinker(BLT_NONE); v.RemoveCommand(CMD_WARNINGLIGHTS_OFF); v.AssignCommand(CMD_WARNINGLIGHTS_ON); } if (v.HasCommand(CMD_FLOODLIGHTS_OFF)) { v.EnableSpecialLights(false); v.RemoveCommand(CMD_FLOODLIGHTS_OFF); v.AssignCommand(CMD_FLOODLIGHTS_ON); } if (v.HasObjectPath(NULL)) Game::ExecuteCommand(DUMMY_PATROL, &v, &v); if (v.HasCommand(DUMMY_HASSIREN) && v.HasCommand(CMD_AUTOSIREN_OFF)) Game::ExecuteCommand(DUMMY_DISABLE, &v, &v); bool ParkinglotFound = false; bool ToDonut = false; ActorList l1; if (!ParkinglotFound) { GameObjectList l2; Game::CollectObstaclesOnVirtualObject(VO_PARK01, l2, ACTOR_VEHICLE); if(l2.GetNumObjects() == 0) { l1 = Game::GetActors(VO_PARK01); ParkinglotFound = true; } } if (!ParkinglotFound) { GameObjectList l3; Game::CollectObstaclesOnVirtualObject(VO_PARK02, l3, ACTOR_VEHICLE); if(l3.GetNumObjects() == 0) { l1 = Game::GetActors(VO_PARK02); ParkinglotFound = true; } } if (!ParkinglotFound) { GameObjectList l4; Game::CollectObstaclesOnVirtualObject(VO_PARK03, l4, ACTOR_VEHICLE); if(l4.GetNumObjects() == 0) { l1 = Game::GetActors(VO_PARK03); ParkinglotFound = true; } } if (Donut && !ParkinglotFound) { GameObjectList l5; Game::CollectObstaclesOnVirtualObject(VO_PARK04, l5, ACTOR_VEHICLE); if (!l5.ContainsSquad()) { l1 = Game::GetActors(VO_PARK04); ParkinglotFound = true; ToDonut = true; } } if (Donut && !ParkinglotFound) { GameObjectList l6; Game::CollectObstaclesOnVirtualObject(VO_PARK05, l6, ACTOR_VEHICLE); if (!l6.ContainsSquad()) { l1 = Game::GetActors(VO_PARK05); ParkinglotFound = true; ToDonut = true; } } if (!ParkinglotFound) { v.PushActionExecuteCommand(ACTION_NEWLIST, CMD_PATROL, Caller, 0, false); return; } else { Vector PD = l1.GetActor(0)->GetPosition(); Vector PD2 = l1.GetActor(0)->GetPosition() + Vector(280,-280,0); ActorList l8 = Game::GetActors(VO_TURNTO); Vector TurnTo = l8.GetActor(0)->GetPosition(); Game::FindFreePosition(Caller, PD); Audio::PlaySample3D(SND_TOSTATION, v.GetPosition()); if (!ToDonut) { v.PushActionMove(ACTION_NEWLIST, PD); v.PushActionTurnTo(ACTION_APPEND, TurnTo); } else { v.PushActionMove(ACTION_NEWLIST, PD2); v.PushActionTurnTo(ACTION_APPEND, PD + Vector(320,-320,0)); v.PushActionWait(ACTION_APPEND, 0.5f); v.PushActionMove(ACTION_APPEND, PD); } v.PushActionWait(ACTION_APPEND, 1.5f); v.PushActionExecuteCommand(ACTION_APPEND, CMD_TOPOLICESTATION, Caller, 2, false); PersonList transports = v.GetTransports(); if (!ToDonut && transports.GetNumPersons() > 0) v.PushActionExecuteCommand(ACTION_APPEND, CMD_TOPOLICESTATION, Caller, 1, false); } } else { Audio::PlaySample3D(SND_TOSTATION, v.GetPosition()); ActorList l1 = Game::GetActors(OBJ_HELIPAD); if(l1.GetNumActors() > 0) { Actor policestation = *l1.GetActor(0); Vector Policestation = policestation.GetPosition(); } if (Game::IsSquadInVirtualObject(VO_HELI)) { GameObjectList l2; Game::CollectObstaclesOnVirtualObject(VO_HELI, l2, ACTOR_VEHICLE); for (int i = 0; i < l2.GetNumObjects(); i++) { Vehicle m = l2.GetObject(i); m.PushActionExecuteCommand(ACTION_NEWLIST, DUMMY_GOHOME, &m, 1, false); Mission::PlayHint(HINT_HELICOPTER); } } Game::FindFreePosition(Caller, Policestation); GameObject obj(&policestation); float landingDirection = v.GetValidLandingAngle(&obj, Policestation); v.PushActionFlyTo(ACTION_NEWLIST, Policestation, true, landingDirection); PersonList transports = v.GetTransports(); if (transports.GetNumPersons() > 0) v.PushActionExecuteCommand(ACTION_APPEND, CMD_TOPOLICESTATION, Caller, 1, false); } } if(ChildID == 1) { if (v.GetVehicleType() != VT_POLICE_PHC) { ActorList l1 = Game::GetActors(VO_SPAWN); if (l1.GetNumActors() > 0) Vector TargetPos = l1.GetActor(0)->GetPosition(); PersonList transports = v.GetTransports(); for(int i = 0; i < transports.GetNumPersons(); i++) { transports.GetPerson(i)->PushActionLeaveCar(ACTION_NEWLIST, Caller); if(transports.GetPerson(i)->GetRole() == ROLE_GANGSTER) { transports.GetPerson(i)->SetRole(ROLE_CIVILIAN); transports.GetPerson(i)->SetBehaviour(BEHAVIOUR_CIVILIAN_NORMAL); } transports.GetPerson(i)->PushActionWait(ACTION_APPEND, 0.5f); transports.GetPerson(i)->PushActionMove(ACTION_APPEND, TargetPos, true); transports.GetPerson(i)->PushActionDeleteOwner(ACTION_APPEND); } } else { ActorList l1 = Game::GetActors(VO_ENTRY_HELIPAD); if (l1.GetNumActors() > 0) Vector TargetPos = l1.GetActor(0)->GetPosition(); PersonList transports = v.GetTransports(); for(int i = 0; i < transports.GetNumPersons(); i++) { transports.GetPerson(i)->PushActionLeaveCar(ACTION_NEWLIST, Caller); if(transports.GetPerson(i)->GetRole() == ROLE_GANGSTER) { transports.GetPerson(i)->SetRole(ROLE_CIVILIAN); transports.GetPerson(i)->SetBehaviour(BEHAVIOUR_CIVILIAN_NORMAL); } transports.GetPerson(i)->PushActionWait(ACTION_APPEND, 0.5f); transports.GetPerson(i)->PushActionMove(ACTION_APPEND, TargetPos, true); transports.GetPerson(i)->PushActionDeleteOwner(ACTION_APPEND); } } } if(ChildID == 2) { Vehicle v(Caller); v.EnableBlinker(BLT_NONE); //disable headlights test v.EnableHeadLights(false); //end test bool ToPoliceStation = false; if (v.IsCollidingWithVirtualObject(VO_PARK01)) return; else ToPoliceStation = true; if (v.IsCollidingWithVirtualObject(VO_PARK02)) return; else ToPoliceStation = true; if (v.IsCollidingWithVirtualObject(VO_PARK03)) return; else ToPoliceStation = true; if (v.IsCollidingWithVirtualObject(VO_PARK04)) return; else ToPoliceStation = true; if (v.IsCollidingWithVirtualObject(VO_PARK05)) return; else ToPoliceStation = true; if(ToPoliceStation) v.PushActionExecuteCommand(ACTION_NEWLIST, CMD_TOPOLICESTATION, Caller, 0, false); } } }; Do You see any problems? and what map was i sposed to edit i edited dfreeplay
-
LA Mod 4x4 w00ds Map v1 Submod Preview
firefighter243 replied to Xplorer4x4's topic in Submods [Not released]
Well...one is better than none -
U.S. Coast Guard & National Guard Outposts
firefighter243 replied to Agentchung's topic in Alteration Help
just like the police station scirt only you would have to make a new scirt -
ok new problem when i edited the scrits it says in the game that there is a scirt error and then NO polic car can go to the station any ideas (sorry for double post)
-
thanks ALOT i will upload the scirpts as soon i i finsh them and make sure they work
-
once i get them working i will most likey make a FBI base and a technal base and a spot for ablances at the hospital parking lot and so on and so forth but again i cant save the scirts that is the only problem.
-
Will THESE computer work with these games ? (4 PC'S)
firefighter243 replied to FDNYEMS22's topic in Technical Related Support
Gta IV Will work as long as you have 2.5(depending if you have vista or windows 7) it sould work with the right video card. A 256MB NVidia 7900 / 256MB ATI X1900 512MB NVIDIA 8600 / 512MB ATI 3870 or better will work -
I am only good with lights but no light bars just lights
-
Even More Additional Units+AMR Ambulance
firefighter243 replied to jasner's topic in Los Angeles Mod
You will need to download something like "Winzip" or "7-Zip" Personaly 7 zip because it is free but winzip is easyer to use and you have to pay for it -
Hello I have made some changes manly in the police section. I have added a detectives and tried to add more relisism too the game. Note: I have used Xplorer4x4 "LA 4x4Submod"
-
I belive that this requieres alot of scirting for the tow truck and the officer but i have no glue how to do it
-
Do the virtal objects need to be pervectly rectangle or can they be a little off when i go to save the edited scirt it says that it connot find the location of the scirt
-
OK Ilove the mod But when i go to download it the same screen pops up It says it is still loading but no download as happened
-
looking great i hope you release it
-
LA Mod 4x4 w00ds Map v1 Submod Preview
firefighter243 replied to Xplorer4x4's topic in Submods [Not released]
Rubber Bullets -
LA Mod 4x4 w00ds Map v1 Submod Preview
firefighter243 replied to Xplorer4x4's topic in Submods [Not released]
It looks more like a anti-invantry tank we could use it for large riots -
Thanks
-
Would GIMP 2 work to reskin?
-
will GIMP 2 work?
-
LA Mod 4x4 w00ds Map v1 Submod Preview
firefighter243 replied to Xplorer4x4's topic in Submods [Not released]
I Agree maybee they will pull though if not you could try to get someone else to do them mabay some one more trustwerety I would Realy like to see this submod released -
LA Mod 4x4 w00ds Map v1 Submod Preview
firefighter243 replied to Xplorer4x4's topic in Submods [Not released]
Thanks and does California Have State Troopers or is that the CHP? -
LA Mod 4x4 w00ds Map v1 Submod Preview
firefighter243 replied to Xplorer4x4's topic in Submods [Not released]
Does the LASD have a charger? Also does CHP have slick top Potal cars? -
There You go all fixed up
-
Hi I have been in the editor making my game more relitic. First I added Floodlights on the police cars that have them. Then i added floodlights to the ALS fire trucks. here is my website you can see the Floodlights Here. I would also like to add a shot gun to the police officers How can i do this too?